ㅤㅤㅤ
Spring MVC – How to include JS or CSS files in a JSP page 본문
Spring MVC – How to include JS or CSS files in a JSP page
ㅤㅤㅤㅤㅤㅤㅤㅤㅤㅤㅤ 2017. 6. 22. 11:39In this tutorial, we will show you how to include static resources like JavaScript or CSS in a JSP page.
Summary steps :
- Put static resources like cs, js or images into this folder
webapp\resources
- Create a Spring
mvc:resources
mapping - Include in JSP page via JSTL tag
c:url
or Spring tagspring:url
P.S This project is tested with Spring 4.1.6.RELEASE.
1. Project Directory
A standard Maven folder structure, puts the static resources like js and css files into the webapp\resources
folder.
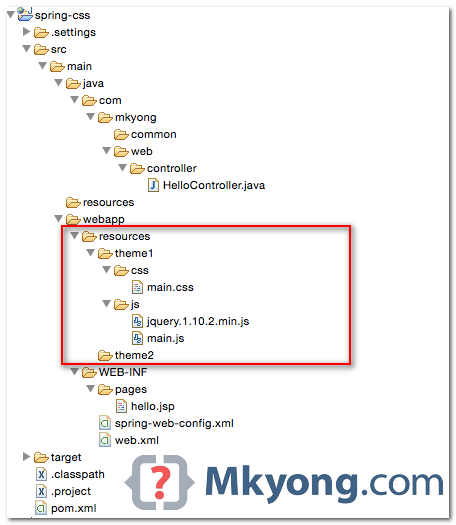
2. Spring Resource Mapping
Declares mvc:resources
, to map “url path” to a physical file path location.
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<context:component-scan base-package="com.mkyong.web" />
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix">
<value>/WEB-INF/pages/</value>
</property>
<property name="suffix">
<value>.jsp</value>
</property>
</bean>
<mvc:resources mapping="/resources/**" location="/resources/theme1/"
cache-period="31556926"/>
<mvc:annotation-driven />
</beans>
In the above example, any requests from this url pattern /resources/**
, Spring will look for the resources from the /resources/mytheme1/
instead.
In future, you can easily change to a new theme by updating the mvc:resources
<mvc:resources mapping="/resources/**" location="/resources/theme-new/" />
3. Include in JSP Page
To include CSS or JS in a JSP page, you can use JSTL tag c:url
or Spring tag spring:url
.
3.1 JSTL tag c:url
example.
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html lang="en">
<head>
<link href="<c:url value="/resources/css/main.css" />" rel="stylesheet">
<script src="<c:url value="/resources/js/jquery.1.10.2.min.js" />"></script>
<script src="<c:url value="/resources/js/main.js" />"></script>
</head>
<body>
<h1>1. Test CSS</h1>
<h2>2. Test JS</h2>
<div id="msg"></div>
</body>
</html>
3.2 Spring tag spring:url
example.
<%@ taglib prefix="spring" uri="http://www.springframework.org/tags"%>
<!DOCTYPE html>
<html lang="en">
<head>
<spring:url value="/resources/css/main.css" var="mainCss" />
<spring:url value="/resources/js/jquery.1.10.2.min.js" var="jqueryJs" />
<spring:url value="/resources/js/main.js" var="mainJs" />
<link href="${mainCss}" rel="stylesheet" />
<script src="${jqueryJs}"></script>
<script src="${mainJs}"></script>
</head>
<body>
<h1>1. Test CSS</h1>
<h2>2. Test JS</h2>
<div id="msg"></div>
</body>
</html>
3.3 Javascript file.
jQuery(document).ready(function($) {
$('#msg').html("This is updated by jQuery")
});
3.4 CSS file.
h1{
color:red;
}
For those who hates JSTL, you can use the “page context” variable to get the resources like this :
<link href="${pageContext.request.contextPath}/resources/css/main.css" rel="stylesheet" >
4. Demo
Run the project with Maven command : $ mvn jetty:run
URL : http://localhost:8080/spring-css/
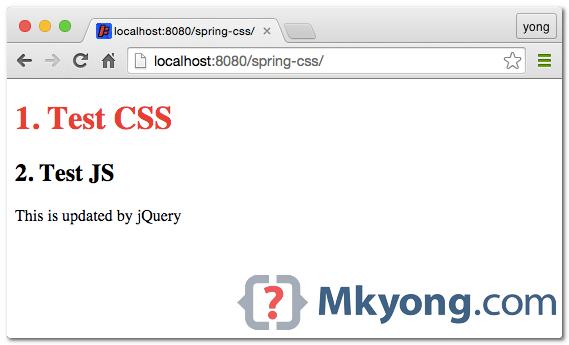
5. Static resources in WEB-INF?
Q : Can I put the static resources in the WEB-INF folder?
A : Yes, you can, the Spring mapping will still work, For example,
<mvc:resources mapping="/resources/**" location="/WEB-INF/resources/theme1/" />
But, this is not a good practice. You can ignore the rule, but many developers and plugins will look for the static resources in the same level of WEB-INF
, not inside the WEB-INF
.